Access Azure SQL DB in .NET Console App
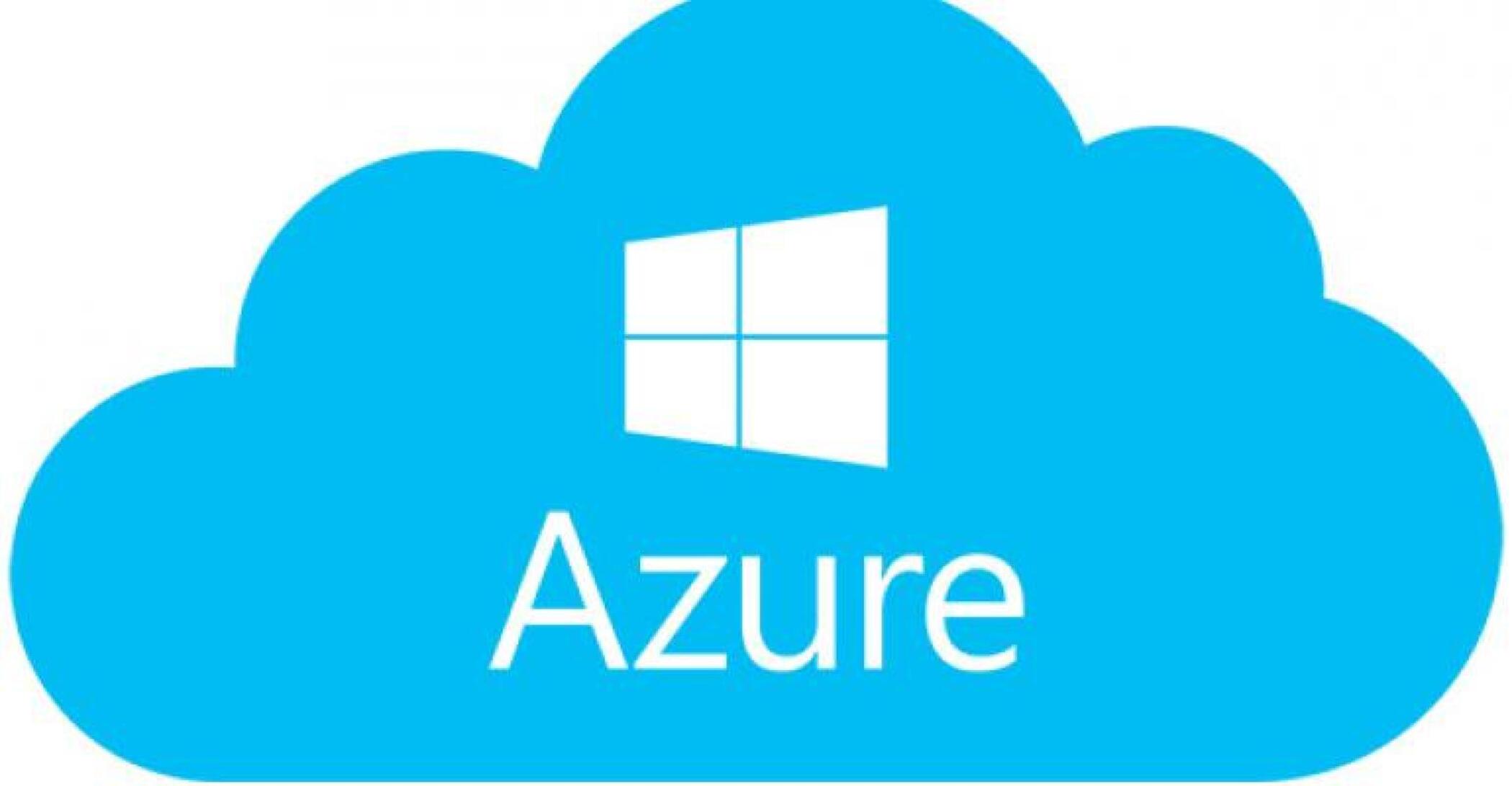
Create a SQL login and user in Azure SQL DB and connect from a simple console app.
Creating a User in Azure SQL DB
Perform the following steps as a DB Admin on the Azure Database in question. Please note that most of these steps come from the following blog entry. I'm re-mentioning them here because sometimes Microsoft Documentation can change without a lot of notice. The Original author is Yochanan Rachamim. His original post can be found here on Tech Community
Step 1: Create SQL Login on Master
CREATE LOGIN <login> WITH PASSWORD = '<strong_password>';
Step 2: Create SQL User on Master Database
use master
go;
CREATE USER <username> FROM LOGIN <login>;
note: username above is normally the same as login.
Step 3: Create SQL User on the Target Database
use {target-database}
go
CREATE USER <username> FROM LOGIN <login>;
note: username above is normally the same as login.
Step 4: Grant permissions to the SQL User on the Target Database
ALTER ROLE db_datareader ADD MEMBER <username>;
Here's a list of SQL Server OOB roles
Connect to the DB via a .NET5 Console App
Using Entity Framework, one technique would be
public const string CONN_STR_PROD = "Server=tcp:{org}.database.windows.net,1433;Database={dbname};User ID={login};Password={password};Trusted_Connection=False;Encrypt=True;";
static void Main(string[] args)
{
var options = new DbContextOptionsBuilder<TriptacularContext>()
.UseSqlServer(CONN_STR_PROD)
.Options;
using (var context = new TriptacularContext(options))
{
context.Database.ExecuteSqlRaw("select name from sys.databases");
}
}